Print ZPL to socket in AL (Business Central OnPrem)
Here you find a snippet to print to a socket directly in Business Central OnPrem or Dynamics NAV (as the DotNet could be run in C/AL as well). This could be helpful if you would like to print to e.g., Zebra Printers using ZPL. For testing purposes, I can recommend the ZPL Test App for Chrome. With this browser-extension you don’t need a physical printer, but only open the port to receive and visualize the data.
To start, we must define the used .NET variables. But don’t forget to update your settings.json first. Otherwise, your VS Code won’t explore the systems assemblies.
{
"al.assemblyProbingPaths": [
"C:/Windows/assembly"
]
}
dotnet
{
assembly(mscorlib)
{
type("System.IO.StreamWriter"; "StreamWriter")
{
}
}
assembly("System")
{
Version = '4.0.0.0';
Culture = 'neutral';
PublicKeyToken = 'b77a5c561934e089';
type("System.Net.Sockets.TcpClient"; "TcpClient")
{
}
type("System.Net.IPAddress"; "IPAddress")
{
}
}
}
Now we could start the actual AL programming and use our defined DotNet assemblies.
It’s just a few lines of code. Open a connection… send the string… done.
[TryFunction]
local procedure PrintZplFileToSocket(IP: Text; Port: Integer; ZPL: Text)
var
IPAddress: DotNet IPAddress;
TcpClient: DotNet TcpClient;
StreamWriter: DotNet StreamWriter;
begin
//Print ZPL to socket
//Parse IP Address
IPAddress := IPAddress.Parse(IP);
//Connect to printer
TcpClient := TcpClient.TcpClient();
TcpClient.Connect(IPAddress, Port);
//Write zpl string to connection
StreamWriter := StreamWriter.StreamWriter(TcpClient.GetStream());
StreamWriter.Write(ZPL);
StreamWriter.Flush();
//Close connection
StreamWriter.Close();
TcpClient.Close();
end;
Finally – Print ZPL to socket
The result is a clean label, directly send from Business Central:
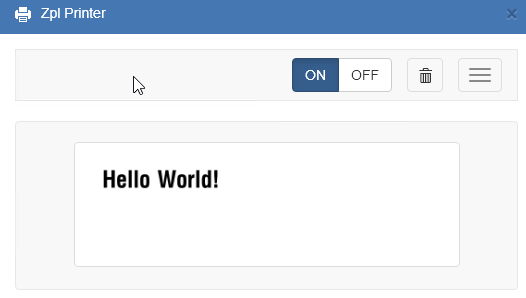
Hello Jens,
thank you very much for sharing the code.
What about the cloud version. Is any solution available there?